What is a Data Transfer Object (DTO)?
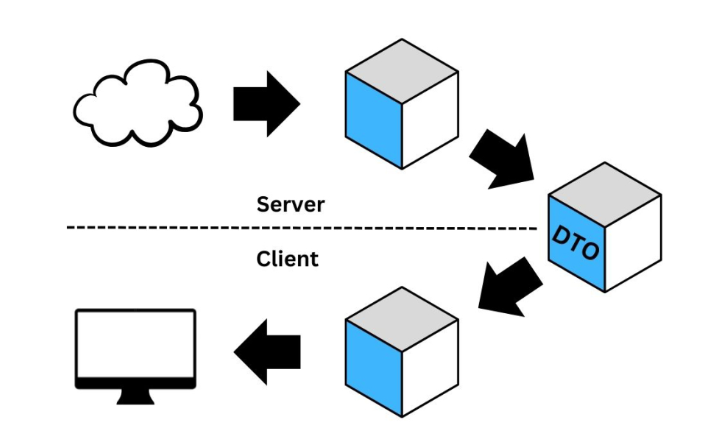
A Data Transfer Object is a simple object that encapsulates data and carries it between processes. DTOs are used to transfer data between software application subsystems. They are not supposed to contain any business logic but only fields for storing data and simple getters/setters.
Why Use DTOs in Laravel REST APIs?
1. Improved Code Readability and Maintainability
DTOs provide a clear contract for data structures, making your code more readable. They define exactly what data is expected, reducing ambiguity and making maintenance easier.
2. Decoupling Between Layers
By using DTOs, you decouple your application’s layers (e.g., Controllers, Services, Repositories). This separation of concerns allows for more flexible code that’s easier to test and modify.
3. Enhanced Data Validation
DTOs can be used to validate data at the point of construction, ensuring that only valid data moves through the system. This leads to more robust applications.
4. Security Benefits
DTOs help prevent over-posting attacks by allowing you to specify exactly which properties can be set, rather than accepting all user input directly into your models.
Integrating DTOs with Models in Laravel
In Laravel, models represent the data from your database. When you use DTOs, you create a layer between your models and controllers. Here’s how you can relate DTOs with models:
1. Data Mapping: Map the data from your DTO to your model attributes.
2. Conversion Methods: Implement methods in your DTO to convert to and from model instances.
3. Repository Pattern: Use repositories to handle data operations, accepting DTOs as parameters.
Using Spatie\DataTransferObject
Spatie\DataTransferObject is a package that provides a convenient way to create DTOs in PHP.
Installation
composer require spatie/data-transfer-object
Defining a DTO
use Spatie\DataTransferObject\DataTransferObject;
class UserData extends DataTransferObject
{
public string $name;
public string $email;
public string $password;
}
Using the DTO in a Controller
use App\DTOs\UserData;
public function store(Request $request)
{
$userData = new UserData($request->all());
// Pass the DTO to a service or repository
$this->userService->createUser($userData);
return response()->json(['message' => 'User created successfully.']);
}
Relating DTOs with Models
use App\Models\User;
class UserService
{
public function createUser(UserData $userData)
{
$user = new User();
$user->name = $userData->name;
$user->email = $userData->email;
$user->password = bcrypt($userData->password);
$user->save();
return $user;
}
}
Why Using Models and DTOs is Not Redundant
Many developers might think that using both models and DTOs is redundant because they both deal with data structures. However, they serve distinct purposes within an application:
• Models: Represent the data structure of your database and handle data persistence, relationships, and business logic related to data storage.
• DTOs: Are simple objects used to transfer data between layers of your application or to external clients. They can include calculated fields or data from external services, which may not be part of your database schema.
By separating these concerns, you achieve:
• Flexibility in Data Representation: DTOs can include only the necessary data for a specific operation, including calculated fields and data from other services.
• Improved Maintainability: Changes in your database schema do not directly impact the DTOs used for API responses or business logic.
• Enhanced Security and Data Control: DTOs allow you to specify exactly which properties are exposed, preventing unintended data leakage.
Example: Logistics Entity with Calculated Fields
Scenario:
Suppose you have a Shipment model representing shipments in your database with fields like id, origin, destination, weight, and created_at. When returning data to clients or other parts of your application, you need to include additional information such as:
• Estimated Delivery Time: A calculated field based on the origin, destination, and current date.
• Current Shipment Status: Information retrieved from an external tracking service.
• Shipping Cost: Calculated based on weight and distance.
Implementing Models and DTOs Together
1. Shipment Model
class Shipment extends Model
{
protected $fillable = ['origin', 'destination', 'weight', 'created_at'];
}
2. ShipmentData DTO
use Spatie\DataTransferObject\DataTransferObject;
class ShipmentData extends DataTransferObject
{
public int $id;
public string $origin;
public string $destination;
public float $weight;
public string $estimated_delivery_time;
public string $current_tracking_status;
public float $shipping_cost;
}
3. Using the DTO in a Controller
use App\Models\Shipment;
use App\DTOs\ShipmentData;
use App\Services\ShippingService;
use App\Services\TrackingService;
public function show($id)
{
$shipment = Shipment::findOrFail($id);
// Calculate the estimated delivery time
$estimatedDeliveryTime = ShippingService::calculateEstimatedDeliveryTime(
$shipment->origin,
$shipment->destination
);
// Get the current tracking status from an external service
$currentTrackingStatus = TrackingService::getCurrentStatus($shipment->id);
// Calculate the shipping cost
$shippingCost = ShippingService::calculateShippingCost(
$shipment->weight,
$shipment->origin,
$shipment->destination
);
// Create the DTO
$shipmentData = new ShipmentData([
'id' => $shipment->id,
'origin' => $shipment->origin,
'destination' => $shipment->destination,
'weight' => $shipment->weight,
'estimated_delivery_time' => $estimatedDeliveryTime,
'current_tracking_status' => $currentTrackingStatus,
'shipping_cost' => $shippingCost,
]);
return response()->json($shipmentData);
}
Explanation:
• Model: Handles data retrieval and interactions with the database.
• DTO: Combines model data, calculated fields, and external service data into a single object for transfer.
Benefits of This Approach:
• Separation of Concerns: Models and DTOs have distinct roles, leading to cleaner code.
• Maintainability: Changes in calculation logic or external services don’t directly affect your database models.
• Data Control: You have explicit control over what data is exposed to the client.
• Code Reusability: DTOs can be reused across different parts of the application or API endpoints.
Advantages of Using Spatie\DataTransferObject
• Type Safety: Ensures that the data types of properties are as expected.
• Immutable Objects: DTOs are immutable by default, preventing unintended side-effects.
• Automatic Validation: Validates the data upon object creation.
Best Practices
• Keep DTOs Simple: They should only contain properties and simple methods like getters.
• Use DTOs for Both Input and Output: Consider using separate DTOs for request data and response data.
• Version Your DTOs: When creating APIs, version your DTOs to handle changes over time.
Conclusion
Incorporating DTO patterns into your Laravel REST API can significantly enhance your application’s structure and maintainability. By decoupling your layers and providing clear data contracts, you make your codebase more robust and easier to work with. Tools like Spatie\DataTransferObject streamline this process, offering additional benefits like type safety and automatic validation.
Keywords: Laravel, DTO, Data Transfer Object, REST API, Spatie\DataTransferObject, Code Structure, Maintainability, Models, PHP, Web Development
By implementing DTOs in your Laravel projects, you’re not only following a best practice but also setting your application up for future success. If you found this article helpful, consider sharing it with your fellow developers!